A radiologist needs to analyze hundreds of medical images daily, and your html5 PACS viewer is their primary tool.
The speed and efficiency of image processing can make or break their workflow.
In this guide, we’ll dive into implementing robust client-side image processing features that will transform your PACS viewer from good to exceptional.
Understanding the Basics
Let’s start with what matters most: the fundamental building blocks of client-side image processing in HTML5. The key components you’ll be working with are:
- Canvas Element: Your primary workspace for image manipulation
- WebGL: The powerhouse for hardware-accelerated processing
- Web Workers: Your solution for handling heavy computations without blocking the UI
Here’s a comparison of different approaches to implement image processing:
Approach | Performance | Browser Support | Complexity | Best For |
Canvas 2D | Moderate | Excellent | Low | Basic manipulations |
WebGL | Excellent | Good | High | Complex processing |
Web Workers | Good | Excellent | Moderate | Heavy computations |
Core Image Processing Features
1. Basic Image Manipulation
First, let’s implement the essential features every PACS viewer needs:
class ImageProcessor {
constructor(canvasId) {
this.canvas = document.getElementById(canvasId);
this.ctx = this.canvas.getContext(‘2d’);
}
adjustBrightness(value) {
const imageData = this.ctx.getImageData(0, 0, this.canvas.width, this.canvas.height);
const data = imageData.data;
for (let i = 0; i < data.length; i += 4) {
data[i] += value; // Red
data[i + 1] += value; // Green
data[i + 2] += value; // Blue
}
this.ctx.putImageData(imageData, 0, 0);
}
}
Key features to implement include:
- Window/Level adjustments
- Pan and zoom
- Rotation and flip
- Basic measurements
2. Advanced Processing
Now, let’s tackle more sophisticated processing techniques:
const sharpnessKernel = [
0, -1, 0,
-1, 5, -1,
0, -1, 0
];
function applyConvolutionFilter(imageData, kernel) {
// Implementation details…
}
Performance Optimization
Your PACS viewer needs to handle large datasets efficiently. Here are the key metrics you should aim for:
Operation | Target Time | Optimization Technique |
Image Load | < 100ms | Progressive loading |
Pan/Zoom | < 16ms | WebGL acceleration |
Filters | < 50ms | Web Workers |
Series Stack | < 200ms | Preloading |
Implementing WebGL Acceleration
Here’s a performance-optimized approach using WebGL:
const vertexShaderSource = `
attribute vec2 a_position;
attribute vec2 a_texCoord;
varying vec2 v_texCoord;
void main() {
gl_Position = vec4(a_position, 0, 1);
v_texCoord = a_texCoord;
}
`;
const fragmentShaderSource = `
precision mediump float;
uniform sampler2D u_image;
uniform float u_brightness;
varying vec2 v_texCoord;
void main() {
vec4 color = texture2D(u_image, v_texCoord);
gl_FragColor = vec4(color.rgb + u_brightness, color.a);
}
`;
Best practices for optimization:
- Use texture atlases for a series of images
- Implement progressive loading for extensive studies
- Optimize memory usage with proper disposal
- Cache processed results
Advanced Techniques
Let’s explore some advanced features that will set your viewer apart:
1. Real-time Filters
Implement sophisticated image processing filters:
class AdvancedImageProcessor extends ImageProcessor {
applyAdaptiveHistogram() {
const imageData = this.ctx.getImageData(0, 0, this.canvas.width, this.canvas.height);
// CLAHE implementation…
return processedImageData;
}
}
2. 3D MPR Support
Multi-planar reconstruction requires careful handling:
class MPRProcessor {
constructor(volumeData) {
this.volume = volumeData;
this.planes = {
axial: new Float32Array(/* … */),
sagittal: new Float32Array(/* … */),
coronal: new Float32Array(/* … */)
};
}
}
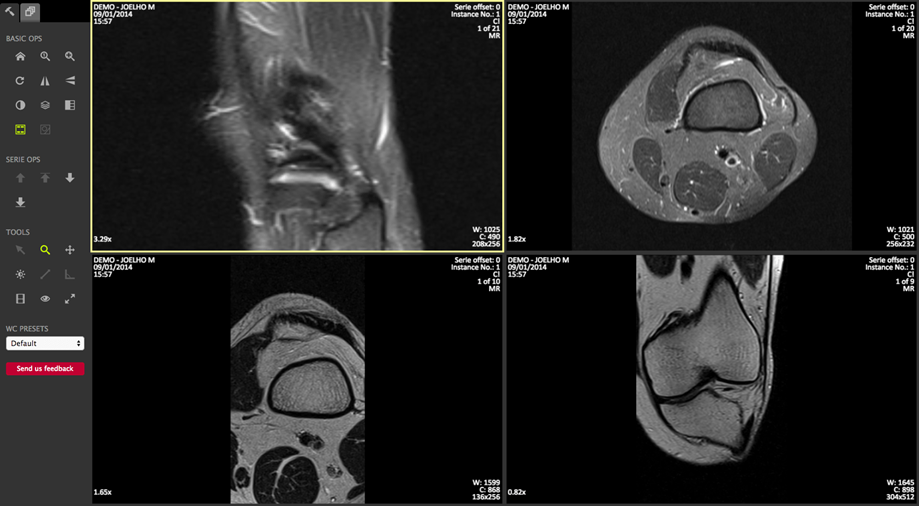
Implementation Best Practices
Follow these guidelines to ensure your implementation is robust and maintainable:
Error Handling
function loadDicomImage(buffer) {
try {
// Implementation
} catch (error) {
console.error(‘DICOM loading failed:’, error);
throw new DicomLoadError(error.message);
}
}
- Memory Management
class ResourceManager {
constructor(maxMemoryMB) {
this.maxMemory = maxMemoryMB * 1024 * 1024;
this.currentUsage = 0;
}
allocateMemory(bytes) {
if (this.currentUsage + bytes > this.maxMemory) {
this.clearCache();
}
this.currentUsage += bytes;
}
}
- Performance Benchmarks
Here’s what you should expect in terms of performance:
Feature | Desktop | Mobile | Memory Usage |
Load 100 Images | 2-3s | 4-6s | ~500MB |
Apply Filter | 50ms | 100ms | +20MB |
MPR Generation | 200ms | 400ms | +100MB |
Stack Scrolling | 16ms | 32ms | Negligible |
This comprehensive guide covers essential techniques for implementing powerful client-side image processing features in your HTML5 PACS viewer.